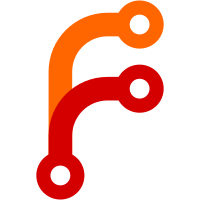
* refactor: clean up webFrame implementation to use gin wrappers The previous implementation of webFrame in the renderer process leaked sub-frame contexts and global objects across the context boundaries thus making it possible for apps to either maliciously or accidentally violate the contextIsolation boundary. This re-implementation binds all methods in native code directly to content::RenderFrame instances instead of relying on JS to provide a "window" with every method request. This is much more consistent with the rest of the Electron codebase and is substantially safer. * chore: un-re-order for ease of review * chore: pass isolate around instead of ErrorThrower * chore: fix rebase typo * chore: remove unused variables
35 lines
1.3 KiB
TypeScript
35 lines
1.3 KiB
TypeScript
const { mainFrame } = process._linkedBinding('electron_renderer_web_frame');
|
|
const binding = process._linkedBinding('electron_renderer_context_bridge');
|
|
|
|
const contextIsolationEnabled = mainFrame.getWebPreference('contextIsolation');
|
|
|
|
const checkContextIsolationEnabled = () => {
|
|
if (!contextIsolationEnabled) throw new Error('contextBridge API can only be used when contextIsolation is enabled');
|
|
};
|
|
|
|
const contextBridge: Electron.ContextBridge = {
|
|
exposeInMainWorld: (key: string, api: any) => {
|
|
checkContextIsolationEnabled();
|
|
return binding.exposeAPIInMainWorld(key, api);
|
|
}
|
|
};
|
|
|
|
export default contextBridge;
|
|
|
|
export const internalContextBridge = {
|
|
contextIsolationEnabled,
|
|
overrideGlobalValueFromIsolatedWorld: (keys: string[], value: any) => {
|
|
return binding._overrideGlobalValueFromIsolatedWorld(keys, value, false);
|
|
},
|
|
overrideGlobalValueWithDynamicPropsFromIsolatedWorld: (keys: string[], value: any) => {
|
|
return binding._overrideGlobalValueFromIsolatedWorld(keys, value, true);
|
|
},
|
|
overrideGlobalPropertyFromIsolatedWorld: (keys: string[], getter: Function, setter?: Function) => {
|
|
return binding._overrideGlobalPropertyFromIsolatedWorld(keys, getter, setter || null);
|
|
},
|
|
isInMainWorld: () => binding._isCalledFromMainWorld() as boolean
|
|
};
|
|
|
|
if (binding._isDebug) {
|
|
contextBridge.internalContextBridge = internalContextBridge;
|
|
}
|