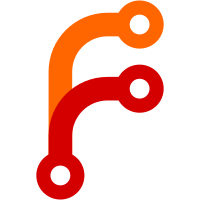
Currently there is no way to know the devtools's state in brightray, so we have to record the state manually, fix this after patching brightray.
114 lines
2.6 KiB
JavaScript
114 lines
2.6 KiB
JavaScript
var app = require('app');
|
|
var delegate = require('atom_delegate');
|
|
var ipc = require('ipc');
|
|
var Menu = require('menu');
|
|
var MenuItem = require('menu_item');
|
|
var BrowserWindow = require('browser_window');
|
|
|
|
var mainWindow = null;
|
|
var menu = null;
|
|
|
|
// Quit when all windows are closed.
|
|
app.on('window-all-closed', function() {
|
|
app.terminate();
|
|
});
|
|
|
|
delegate.browserMainParts.preMainMessageLoopRun = function() {
|
|
app.commandLine.appendSwitch('js-flags', '--harmony_collections');
|
|
|
|
mainWindow = new BrowserWindow({ width: 800, height: 600 });
|
|
mainWindow.loadUrl('file://' + __dirname + '/index.html');
|
|
|
|
mainWindow.on('page-title-updated', function(event, title) {
|
|
event.preventDefault();
|
|
|
|
this.setTitle('Atom Shell - ' + title);
|
|
});
|
|
|
|
mainWindow.on('closed', function() {
|
|
console.log('closed');
|
|
mainWindow = null;
|
|
});
|
|
|
|
var template = [
|
|
{
|
|
label: 'Atom Shell',
|
|
submenu: [
|
|
{
|
|
label: 'About Atom Shell',
|
|
selector: 'orderFrontStandardAboutPanel:'
|
|
},
|
|
{
|
|
type: 'separator'
|
|
},
|
|
{
|
|
label: 'Hide Atom Shell',
|
|
accelerator: 'Command+H',
|
|
selector: 'hide:'
|
|
},
|
|
{
|
|
label: 'Hide Others',
|
|
accelerator: 'Command+Shift+H',
|
|
selector: 'hideOtherApplications:'
|
|
},
|
|
{
|
|
label: 'Show All',
|
|
selector: 'unhideAllApplications:'
|
|
},
|
|
{
|
|
type: 'separator'
|
|
},
|
|
{
|
|
label: 'Quit',
|
|
accelerator: 'Command+Q',
|
|
click: function() { app.quit(); }
|
|
},
|
|
]
|
|
},
|
|
{
|
|
label: 'View',
|
|
submenu: [
|
|
{
|
|
label: 'Reload',
|
|
accelerator: 'Command+R',
|
|
click: function() { BrowserWindow.getFocusedWindow().reloadIgnoringCache(); }
|
|
},
|
|
{
|
|
label: 'Toggle DevTools',
|
|
accelerator: 'Alt+Command+I',
|
|
click: function() { BrowserWindow.getFocusedWindow().toggleDevTools(); }
|
|
},
|
|
]
|
|
},
|
|
{
|
|
label: 'Window',
|
|
submenu: [
|
|
{
|
|
label: 'Minimize',
|
|
accelerator: 'Command+M',
|
|
selector: 'performMiniaturize:'
|
|
},
|
|
{
|
|
label: 'Close',
|
|
accelerator: 'Command+W',
|
|
selector: 'performClose:'
|
|
},
|
|
{
|
|
type: 'separator'
|
|
},
|
|
{
|
|
label: 'Bring All to Front',
|
|
selector: 'arrangeInFront:'
|
|
},
|
|
]
|
|
},
|
|
];
|
|
|
|
menu = Menu.buildFromTemplate(template);
|
|
Menu.setApplicationMenu(menu);
|
|
|
|
ipc.on('message', function(processId, routingId, type) {
|
|
if (type == 'menu')
|
|
menu.popup(mainWindow);
|
|
});
|
|
}
|