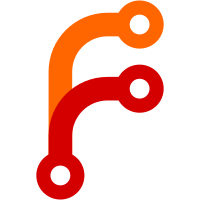
* refactor: desttroy URLRequestContextGetter on IO thread * Accepts a factory class that can customize the creation of URLRequestContext * Use a separate request context for media which is derived from the default * Notify URLRequestContextGetter observers and cleanup on IO thread * Move most of brightray net/ classes into atom net/ * refactor: remove refs to URLRequestContextGetter on shutdown * refactor: remove brigtray switches.{cc|h} * refactor: remove brightray network_delegate.{cc|h} * refactor: make AtomURLRequestJobFactory the top level factory. * Allows to use the default handler from content/ for http{s}, ws{s} schemes. * Removes the storage of job factory in URLRequestContextGetter.
82 lines
2.5 KiB
C++
82 lines
2.5 KiB
C++
// Copyright (c) 2012 The Chromium Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style license that can be
|
|
// found in the LICENSE-CHROMIUM file.
|
|
|
|
#ifndef BRIGHTRAY_BROWSER_URL_REQUEST_CONTEXT_GETTER_H_
|
|
#define BRIGHTRAY_BROWSER_URL_REQUEST_CONTEXT_GETTER_H_
|
|
|
|
#include "brightray/browser/net/url_request_context_getter_factory.h"
|
|
#include "content/public/browser/resource_context.h"
|
|
#include "net/url_request/url_request_context.h"
|
|
#include "net/url_request/url_request_context_getter.h"
|
|
|
|
#if DCHECK_IS_ON()
|
|
#include "base/debug/leak_tracker.h"
|
|
#endif
|
|
|
|
namespace brightray {
|
|
|
|
class BrowserContext;
|
|
class ResourceContext;
|
|
|
|
class URLRequestContextGetter : public net::URLRequestContextGetter {
|
|
public:
|
|
URLRequestContextGetter(URLRequestContextGetterFactory* factory,
|
|
ResourceContext* resource_context);
|
|
|
|
// net::URLRequestContextGetter:
|
|
net::URLRequestContext* GetURLRequestContext() override;
|
|
scoped_refptr<base::SingleThreadTaskRunner> GetNetworkTaskRunner()
|
|
const override;
|
|
|
|
// Discard reference to URLRequestContext and inform observers to
|
|
// shutdown. Must be called only on IO thread.
|
|
void NotifyContextShuttingDown();
|
|
|
|
private:
|
|
friend class BrowserContext;
|
|
|
|
// Responsible for destroying URLRequestContextGetter
|
|
// on the IO thread.
|
|
class Handle {
|
|
public:
|
|
explicit Handle(base::WeakPtr<BrowserContext> browser_context);
|
|
~Handle();
|
|
|
|
scoped_refptr<URLRequestContextGetter> CreateMainRequestContextGetter(
|
|
URLRequestContextGetterFactory* factory);
|
|
content::ResourceContext* GetResourceContext() const;
|
|
scoped_refptr<URLRequestContextGetter> GetMediaRequestContextGetter() const;
|
|
|
|
void ShutdownOnUIThread();
|
|
|
|
private:
|
|
void LazyInitialize() const;
|
|
|
|
scoped_refptr<URLRequestContextGetter> main_request_context_getter_;
|
|
scoped_refptr<URLRequestContextGetter> media_request_context_getter_;
|
|
std::unique_ptr<ResourceContext> resource_context_;
|
|
base::WeakPtr<BrowserContext> browser_context_;
|
|
mutable bool initialized_;
|
|
|
|
DISALLOW_COPY_AND_ASSIGN(Handle);
|
|
};
|
|
|
|
~URLRequestContextGetter() override;
|
|
|
|
#if DCHECK_IS_ON()
|
|
base::debug::LeakTracker<URLRequestContextGetter> leak_tracker_;
|
|
#endif
|
|
|
|
std::unique_ptr<URLRequestContextGetterFactory> factory_;
|
|
|
|
ResourceContext* resource_context_;
|
|
net::URLRequestContext* url_request_context_;
|
|
bool context_shutting_down_;
|
|
|
|
DISALLOW_COPY_AND_ASSIGN(URLRequestContextGetter);
|
|
};
|
|
|
|
} // namespace brightray
|
|
|
|
#endif // BRIGHTRAY_BROWSER_URL_REQUEST_CONTEXT_GETTER_H_
|