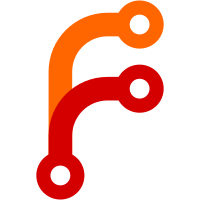
When creating menus, the accelerators must be converted to platform accelerators before they can be used.
34 lines
1.3 KiB
Text
34 lines
1.3 KiB
Text
// Copyright (c) 2013 GitHub, Inc. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#include "browser/accelerator_util.h"
|
|
|
|
#include "ui/base/accelerators/accelerator.h"
|
|
#import "ui/base/accelerators/platform_accelerator_cocoa.h"
|
|
#import "ui/base/keycodes/keyboard_code_conversion_mac.h"
|
|
|
|
namespace accelerator_util {
|
|
|
|
void SetPlatformAccelerator(ui::Accelerator* accelerator) {
|
|
unichar character;
|
|
unichar characterIgnoringModifiers;
|
|
ui::MacKeyCodeForWindowsKeyCode(accelerator->key_code(),
|
|
0,
|
|
&character,
|
|
&characterIgnoringModifiers);
|
|
NSString* characters =
|
|
[[[NSString alloc] initWithCharacters:&character length:1] autorelease];
|
|
|
|
NSUInteger modifiers =
|
|
(accelerator->IsCtrlDown() ? NSControlKeyMask : 0) |
|
|
(accelerator->IsCmdDown() ? NSCommandKeyMask : 0) |
|
|
(accelerator->IsAltDown() ? NSAlternateKeyMask : 0) |
|
|
(accelerator->IsShiftDown() ? NSShiftKeyMask : 0);
|
|
|
|
scoped_ptr<ui::PlatformAccelerator> platform_accelerator(
|
|
new ui::PlatformAcceleratorCocoa(characters, modifiers));
|
|
accelerator->set_platform_accelerator(platform_accelerator.Pass());
|
|
}
|
|
|
|
} // namespace accelerator_util
|