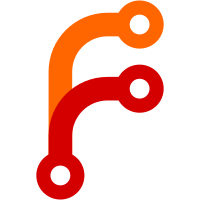
* refactor: convert Menu and globalShortcut to gin * refactor: convert api::Cookies to gin * refactor: convert View and WebContentsView to gin * refactor: convert WebContents related classes to gin * refactor: convert powerMonitor to gin * refactor: prepare for header change * refactor: remove last uses of mate::EventEmitter * refactor: remove mate::EventEmitter * refactor: move trackable_object to gin_helper * fix: custom converter should not use Handle * fix: no more need to check if icon is empty It was a bug that the Handle<NativeImage> can be non-empty when the image file does not exist. The bug was caused by the converter code writing out the image even when the convertion fails. The bug was work-arounded by adding an additional check, but since the original bug had been fixed, the additional check is no longer needed. * fix: should always set frameId even when callback is null * fix: do not mix gin/mate handles for NativeImage
79 lines
2.4 KiB
C++
79 lines
2.4 KiB
C++
// Copyright (c) 2019 GitHub, Inc.
|
|
// Use of this source code is governed by the MIT license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#ifndef SHELL_COMMON_GIN_CONVERTERS_BLINK_CONVERTER_GIN_ADAPTER_H_
|
|
#define SHELL_COMMON_GIN_CONVERTERS_BLINK_CONVERTER_GIN_ADAPTER_H_
|
|
|
|
#include "gin/converter.h"
|
|
#include "shell/common/native_mate_converters/blink_converter.h"
|
|
|
|
// TODO(zcbenz): Move the implementations from native_mate_converters to here.
|
|
|
|
namespace gin {
|
|
|
|
template <>
|
|
struct Converter<blink::WebKeyboardEvent> {
|
|
static bool FromV8(v8::Isolate* isolate,
|
|
v8::Local<v8::Value> val,
|
|
blink::WebKeyboardEvent* out) {
|
|
return mate::ConvertFromV8(isolate, val, out);
|
|
}
|
|
};
|
|
|
|
template <>
|
|
struct Converter<blink::CloneableMessage> {
|
|
static bool FromV8(v8::Isolate* isolate,
|
|
v8::Local<v8::Value> val,
|
|
blink::CloneableMessage* out) {
|
|
return mate::ConvertFromV8(isolate, val, out);
|
|
}
|
|
static v8::Local<v8::Value> ToV8(v8::Isolate* isolate,
|
|
const blink::CloneableMessage& val) {
|
|
return mate::ConvertToV8(isolate, val);
|
|
}
|
|
};
|
|
|
|
template <>
|
|
struct Converter<blink::WebDeviceEmulationParams> {
|
|
static bool FromV8(v8::Isolate* isolate,
|
|
v8::Local<v8::Value> val,
|
|
blink::WebDeviceEmulationParams* out) {
|
|
return mate::ConvertFromV8(isolate, val, out);
|
|
}
|
|
};
|
|
|
|
template <>
|
|
struct Converter<blink::WebContextMenuData::MediaType> {
|
|
static v8::Local<v8::Value> ToV8(
|
|
v8::Isolate* isolate,
|
|
const blink::WebContextMenuData::MediaType& in) {
|
|
return mate::ConvertToV8(isolate, in);
|
|
}
|
|
};
|
|
|
|
template <>
|
|
struct Converter<blink::WebContextMenuData::InputFieldType> {
|
|
static v8::Local<v8::Value> ToV8(
|
|
v8::Isolate* isolate,
|
|
const blink::WebContextMenuData::InputFieldType& in) {
|
|
return mate::ConvertToV8(isolate, in);
|
|
}
|
|
};
|
|
|
|
template <>
|
|
struct Converter<network::mojom::ReferrerPolicy> {
|
|
static v8::Local<v8::Value> ToV8(v8::Isolate* isolate,
|
|
const network::mojom::ReferrerPolicy& in) {
|
|
return mate::ConvertToV8(isolate, in);
|
|
}
|
|
static bool FromV8(v8::Isolate* isolate,
|
|
v8::Local<v8::Value> val,
|
|
network::mojom::ReferrerPolicy* out) {
|
|
return mate::ConvertFromV8(isolate, val, out);
|
|
}
|
|
};
|
|
|
|
} // namespace gin
|
|
|
|
#endif // SHELL_COMMON_GIN_CONVERTERS_BLINK_CONVERTER_GIN_ADAPTER_H_
|