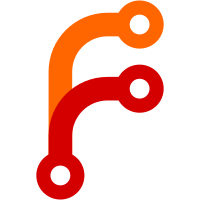
Privously we release them when the window is unloaded, which is not correct since a render view can have multiple windows (or js contexts) and when the unload event is emitted the render view could already have gone. This PR does the cleaning work purely in browser, so here is no need to worry about renderer's life time.
47 lines
1.3 KiB
C++
47 lines
1.3 KiB
C++
// Copyright (c) 2013 GitHub, Inc. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style license that can be
|
|
// found in the LICENSE file.
|
|
|
|
#ifndef ATOM_BROWSER_NATIVE_WINDOW_OBSERVER_H_
|
|
#define ATOM_BROWSER_NATIVE_WINDOW_OBSERVER_H_
|
|
|
|
#include <string>
|
|
|
|
namespace atom {
|
|
|
|
class NativeWindowObserver {
|
|
public:
|
|
virtual ~NativeWindowObserver() {}
|
|
|
|
// Called when the web page of the window has updated it's document title.
|
|
virtual void OnPageTitleUpdated(bool* prevent_default,
|
|
const std::string& title) {}
|
|
|
|
// Called when the window is starting or is done loading a page.
|
|
virtual void OnLoadingStateChanged(bool is_loading) {}
|
|
|
|
// Called when the window is gonna closed.
|
|
virtual void WillCloseWindow(bool* prevent_default) {}
|
|
|
|
// Called when the window is closed.
|
|
virtual void OnWindowClosed() {}
|
|
|
|
// Called when window loses focus.
|
|
virtual void OnWindowBlur() {}
|
|
|
|
// Called when renderer is hung.
|
|
virtual void OnRendererUnresponsive() {}
|
|
|
|
// Called when renderer recovers.
|
|
virtual void OnRendererResponsive() {}
|
|
|
|
// Called when a render view has been deleted.
|
|
virtual void OnRenderViewDeleted() {}
|
|
|
|
// Called when renderer has crashed.
|
|
virtual void OnRendererCrashed() {}
|
|
};
|
|
|
|
} // namespace atom
|
|
|
|
#endif // ATOM_BROWSER_NATIVE_WINDOW_OBSERVER_H_
|