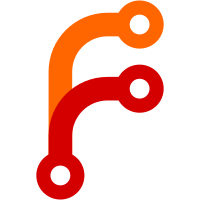
- Added PackOptions, RuntimeOptions, PublishOptions and updated CompilationOptions - Added IncludeFilesResolver to parse include, exclude patterns - Added compile, embed and copyToOutput to compilationOptions - Renamed compilationOptions to buildOptions - Moved compilerName into buildOptions - This change is backwards compatible - Added warnings to be shown when the old schema is used - Handled diagnostic messages in ProjectReader - Added unit and end to end tests
32 lines
1,021 B
C#
32 lines
1,021 B
C#
// Copyright (c) .NET Foundation and contributors. All rights reserved.
|
|
// Licensed under the MIT license. See LICENSE file in the project root for full license information.
|
|
|
|
using System;
|
|
using System.Collections.Generic;
|
|
using System.Linq;
|
|
using System.Threading.Tasks;
|
|
|
|
namespace Microsoft.DotNet.ProjectModel
|
|
{
|
|
public static class ProjectExtensions
|
|
{
|
|
private static readonly KeyValuePair<string, string>[] _compilerNameToLanguageId =
|
|
{
|
|
new KeyValuePair<string, string>("csc", "cs"),
|
|
new KeyValuePair<string, string>("vbc", "vb"),
|
|
new KeyValuePair<string, string>("fsc", "fs")
|
|
};
|
|
|
|
public static string GetSourceCodeLanguage(this Project project)
|
|
{
|
|
foreach (var kvp in _compilerNameToLanguageId)
|
|
{
|
|
if (kvp.Key == (project._defaultCompilerOptions.CompilerName))
|
|
{
|
|
return kvp.Value;
|
|
}
|
|
}
|
|
return null;
|
|
}
|
|
}
|
|
}
|