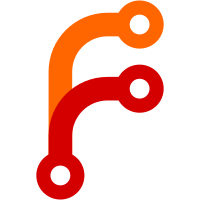
- Needs a clean machine without dotnet MSI installed for the tests to run. - Needs admin privileges to run. Else test script exits silently. - These xunit based tests run on Netfx46. For now these tests are disabled until I figure out the right way to run them in the CI machines.
27 lines
806 B
C#
27 lines
806 B
C#
using Microsoft.Win32;
|
|
using System;
|
|
using System.Collections.Generic;
|
|
using System.IO;
|
|
using System.Linq;
|
|
|
|
namespace Dotnet.Cli.Msi.Tests
|
|
{
|
|
class Utils
|
|
{
|
|
internal static bool ExistsOnPath(string fileName)
|
|
{
|
|
var paths = GetCurrentPathEnvironmentVariable();
|
|
return paths
|
|
.Split(';')
|
|
.Any(path => File.Exists(Path.Combine(path, fileName)));
|
|
}
|
|
|
|
internal static string GetCurrentPathEnvironmentVariable()
|
|
{
|
|
var hklm = RegistryKey.OpenBaseKey(RegistryHive.LocalMachine, RegistryView.Registry32);
|
|
var regKey = hklm.OpenSubKey(@"SYSTEM\CurrentControlSet\Control\Session Manager\Environment", false);
|
|
|
|
return (string)regKey.GetValue("Path");
|
|
}
|
|
}
|
|
}
|