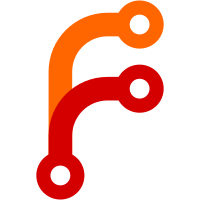
For injestion into a composed build, I'm adding some minor tweaks to our build system: - Teach DownloadFile task how to consume file:// URLs so we can pass around artifacts using local sources. - Add `SkipBuildingInstallers`, an MSBuild property that can be set to true when installers (pkgs, msis, debs, rpms and the like) do not need to be built. - Add `IncludeAdditionalSharedFrameworks`, an MSBuild property that can be set to prevent additional shared frameworks (i.e. shared frameworks that the CLI does not use at runtime) from being downloaded and included in our payload. - Add `IncludeNuGetPackageArchive` an MSBuild property that can be set to prevent the lzma archive containing all the nupkgs we restore on first run from being included in the final output. - Provide a way to change the Uri use for blob storage (so the composed build and point at a local folder that looks like blob storage to pick up artifacts from).
65 lines
1.9 KiB
C#
65 lines
1.9 KiB
C#
// Copyright (c) .NET Foundation and contributors. All rights reserved.
|
|
// Licensed under the MIT license. See LICENSE file in the project root for full license information.
|
|
|
|
using System;
|
|
using System.IO;
|
|
using System.Net.Http;
|
|
using Microsoft.Build.Framework;
|
|
using Microsoft.Build.Utilities;
|
|
|
|
namespace Microsoft.DotNet.Cli.Build
|
|
{
|
|
public class DownloadFile : Task
|
|
{
|
|
[Required]
|
|
public string Uri { get; set; }
|
|
|
|
[Required]
|
|
public string DestinationPath { get; set; }
|
|
|
|
public bool Overwrite { get; set; }
|
|
|
|
public override bool Execute()
|
|
{
|
|
FS.Mkdirp(Path.GetDirectoryName(DestinationPath));
|
|
|
|
if (File.Exists(DestinationPath) && !Overwrite)
|
|
{
|
|
return true;
|
|
}
|
|
|
|
const string FileUriProtocol = "file://";
|
|
|
|
if (Uri.StartsWith(FileUriProtocol, StringComparison.Ordinal))
|
|
{
|
|
var filePath = Uri.Substring(FileUriProtocol.Length);
|
|
Log.LogMessage($"Copying '{filePath}' to '{DestinationPath}'");
|
|
File.Copy(filePath, DestinationPath);
|
|
}
|
|
else
|
|
{
|
|
Log.LogMessage($"Downloading '{Uri}' to '{DestinationPath}'");
|
|
|
|
using (var httpClient = new HttpClient())
|
|
{
|
|
var getTask = httpClient.GetStreamAsync(Uri);
|
|
|
|
try
|
|
{
|
|
using (var outStream = File.Create(DestinationPath))
|
|
{
|
|
getTask.Result.CopyTo(outStream);
|
|
}
|
|
}
|
|
catch (Exception)
|
|
{
|
|
File.Delete(DestinationPath);
|
|
throw;
|
|
}
|
|
}
|
|
}
|
|
|
|
return true;
|
|
}
|
|
}
|
|
}
|