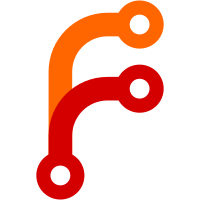
* compose all the parts * Fix on obtain and shim maker for better end to end experience * Fix error when there is space in the middle of path of nuget config * Fix path in profile.d is the tmp home path during install * better handle of ~home * remove profile.d file in uninstall script * Fix test since it looks up current directory * folder structure inside nupkg to tools/TFM/RID/mytool.dll * Add check for config file existence * Rename name space to Microsoft.DotNet.ShellShim * Rename name space to Microsoft.DotNet.ToolPackage
52 lines
1.8 KiB
C#
52 lines
1.8 KiB
C#
// Copyright (c) .NET Foundation and contributors. All rights reserved.
|
|
// Licensed under the MIT license. See LICENSE file in the project root for full license information.
|
|
|
|
using System.Linq;
|
|
using FluentAssertions;
|
|
using Microsoft.DotNet.Cli;
|
|
using Microsoft.DotNet.Cli.CommandLine;
|
|
using Xunit;
|
|
using Xunit.Abstractions;
|
|
using Parser = Microsoft.DotNet.Cli.Parser;
|
|
|
|
namespace Microsoft.DotNet.Tests.ParserTests
|
|
{
|
|
public class InstallToolParserTests
|
|
{
|
|
private readonly ITestOutputHelper output;
|
|
|
|
public InstallToolParserTests(ITestOutputHelper output)
|
|
{
|
|
this.output = output;
|
|
}
|
|
|
|
[Fact]
|
|
public void InstallGlobaltoolParserCanGetPackageIdAndPackageVersion()
|
|
{
|
|
var command = Parser.Instance;
|
|
var result = command.Parse("dotnet install tool console.test.app --version 1.0.1");
|
|
|
|
var parseResult = result["dotnet"]["install"]["tool"];
|
|
|
|
var packageId = parseResult.Arguments.Single();
|
|
var packageVersion = parseResult.ValueOrDefault<string>("version");
|
|
|
|
packageId.Should().Be("console.test.app");
|
|
packageVersion.Should().Be("1.0.1");
|
|
}
|
|
|
|
[Fact]
|
|
public void InstallGlobaltoolParserCanGetFollowingArguments()
|
|
{
|
|
var command = Parser.Instance;
|
|
var result =
|
|
command.Parse(
|
|
@"dotnet install tool console.test.app --version 1.0.1 --framework netcoreapp2.0 --configfile C:\TestAssetLocalNugetFeed");
|
|
|
|
var parseResult = result["dotnet"]["install"]["tool"];
|
|
|
|
parseResult.ValueOrDefault<string>("configfile").Should().Be(@"C:\TestAssetLocalNugetFeed");
|
|
parseResult.ValueOrDefault<string>("framework").Should().Be("netcoreapp2.0");
|
|
}
|
|
}
|
|
}
|