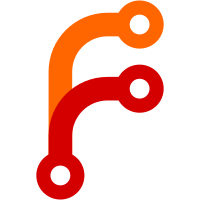
* Partial conversion to new3. 2 tests fail due to browserlink not restoring. * new cache initialization * More lzma changes, and removed a razor ref from templates * Ephemeral hive flag added to tests that need it * Updated the template engine version to build against. Minor code cleanup * Config changes to make template versions separate from template engine versions * Changed dotnet new versioning to use Product.Version * Fixing Archiver.csproj * Fixing dotnet new test. * Fix LZMA Package Source Condition * Workaround for newline differences. * fixed tests with changed template parameters. Added a new3 template non-match test
68 lines
2.5 KiB
C#
68 lines
2.5 KiB
C#
// Copyright (c) .NET Foundation and contributors. All rights reserved.
|
|
// Licensed under the MIT license. See LICENSE file in the project root for full license information.
|
|
|
|
using Microsoft.DotNet.Tools.Test.Utilities;
|
|
using System;
|
|
using System.IO;
|
|
using FluentAssertions;
|
|
using Xunit;
|
|
|
|
namespace Microsoft.DotNet.New.Tests
|
|
{
|
|
public class GivenThatIWantANewApp : TestBase
|
|
{
|
|
[Fact]
|
|
public void When_dotnet_new_is_invoked_mupliple_times_it_should_fail()
|
|
{
|
|
var rootPath = TestAssetsManager.CreateTestDirectory().Path;
|
|
|
|
new TestCommand("dotnet") { WorkingDirectory = rootPath }
|
|
.Execute($"new console --debug:ephemeral-hive");
|
|
|
|
DateTime expectedState = Directory.GetLastWriteTime(rootPath);
|
|
|
|
var result = new TestCommand("dotnet") { WorkingDirectory = rootPath }
|
|
.ExecuteWithCapturedOutput($"new console --debug:ephemeral-hive");
|
|
|
|
DateTime actualState = Directory.GetLastWriteTime(rootPath);
|
|
|
|
Assert.Equal(expectedState, actualState);
|
|
|
|
result.Should().Fail();
|
|
}
|
|
|
|
[Fact]
|
|
public void RestoreDoesNotUseAnyCliProducedPackagesOnItsTemplates()
|
|
{
|
|
string[] cSharpTemplates = new[] { "console", "classlib", "mstest", "xunit", "web", "mvc", "webapi" };
|
|
|
|
var rootPath = TestAssetsManager.CreateTestDirectory().Path;
|
|
var packagesDirectory = Path.Combine(rootPath, "packages");
|
|
|
|
foreach (string cSharpTemplate in cSharpTemplates)
|
|
{
|
|
var projectFolder = Path.Combine(rootPath, cSharpTemplate + "1");
|
|
Directory.CreateDirectory(projectFolder);
|
|
CreateAndRestoreNewProject(cSharpTemplate, projectFolder, packagesDirectory);
|
|
}
|
|
|
|
Directory.EnumerateFiles(packagesDirectory, $"*.nupkg", SearchOption.AllDirectories)
|
|
.Should().NotContain(p => p.Contains("Microsoft.DotNet.Cli.Utils"));
|
|
}
|
|
|
|
private void CreateAndRestoreNewProject(
|
|
string projectType,
|
|
string projectFolder,
|
|
string packagesDirectory)
|
|
{
|
|
new TestCommand("dotnet") { WorkingDirectory = projectFolder }
|
|
.Execute($"new {projectType} --debug:ephemeral-hive")
|
|
.Should().Pass();
|
|
|
|
new RestoreCommand()
|
|
.WithWorkingDirectory(projectFolder)
|
|
.Execute($"--packages {packagesDirectory} /p:SkipInvalidConfigurations=true")
|
|
.Should().Pass();
|
|
}
|
|
}
|
|
}
|