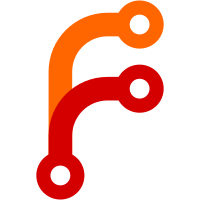
* Create backup folder in the directory where 'dotnet migrate' is executed With this change, 'dotnet migrate' will create the backup folder in the workspace directory rather than the parent of the workspace directory. This solves two problems: 1. It makes it easier for the user where the backup is -- it's in the directory they targeted with 'dotnet migrate'. 2. It solves a problem of file oollisions with global.json files when migrating multiple projects. Consider the following directory structure: root | project1 | global.json | src | project1 project2 | global.json | src | project2 Prior to this change, running 'dotnet migrate' project1 and then running it again in project2 would have caused an exception to be thrown because the migration would try to produce a backup folder like so: root | backup | | | global.json | | | project1 | | | project2 | | project1 | src | project1 project2 | src | project2 Now, we produce the following structure, which has no collisions: root | project1 | backup | | | global.json | | | project1 | src | project1 | project2 | backup | | | global.json | | | project2 | src | project2 In addition, to help avoid further collisions, a number is appened to the backup folder's name if it already exists. So, if the user runs dotnet migrate again for some reason, they'll see backup_1, backup_2, etc. * Fix test helper * Fix foolish bug causing infinite loop * Fix up a couple more tests * Rework MigrationBackupPlan to process all projects at once * Fix up tests * Still fixing tests * Compute common root folder of projects to determine where backup folder should be placed * Fix typo * Fix test to not look in backup folder now that it's in a better location
137 lines
5.3 KiB
C#
137 lines
5.3 KiB
C#
// Copyright (c) .NET Foundation and contributors. All rights reserved.
|
|
// Licensed under the MIT license. See LICENSE file in the project root for full license information.
|
|
|
|
using FluentAssertions;
|
|
using Microsoft.DotNet.Internal.ProjectModel.Utilities;
|
|
using Microsoft.DotNet.ProjectJsonMigration;
|
|
using System;
|
|
using System.Collections.Generic;
|
|
using System.IO;
|
|
using System.Linq;
|
|
using Xunit;
|
|
|
|
namespace Microsoft.DotNet.ProjectJsonMigration.Tests
|
|
{
|
|
public partial class MigrationBackupPlanTests
|
|
{
|
|
[Fact]
|
|
public void TheBackupDirectoryIsASubfolderOfTheMigratedProject()
|
|
{
|
|
var workspaceDirectory = Path.Combine("src", "root");
|
|
var projectDirectory = Path.Combine("src", "project1");
|
|
|
|
WhenMigrating(projectDirectory, workspaceDirectory)
|
|
.RootBackupDirectory
|
|
.FullName
|
|
.Should()
|
|
.Be(new DirectoryInfo(Path.Combine("src", "project1", "backup")).FullName.EnsureTrailingSlash());
|
|
}
|
|
|
|
[Fact]
|
|
public void TheBackupDirectoryIsASubfolderOfTheMigratedProjectWhenInitiatedFromProjectFolder()
|
|
{
|
|
var workspaceDirectory = Path.Combine("src", "root");
|
|
var projectDirectory = Path.Combine("src", "root");
|
|
|
|
WhenMigrating(projectDirectory, workspaceDirectory)
|
|
.ProjectBackupDirectories.Single()
|
|
.FullName
|
|
.Should()
|
|
.Be(new DirectoryInfo(Path.Combine("src", "root", "backup")).FullName.EnsureTrailingSlash());
|
|
}
|
|
|
|
[Fact]
|
|
public void TheBackupDirectoryIsInTheCommonRootOfTwoProjectFoldersWhenInitiatedFromProjectFolder()
|
|
{
|
|
var projectDirectories = new []
|
|
{
|
|
Path.Combine("root", "project1"),
|
|
Path.Combine("root", "project2")
|
|
};
|
|
|
|
var workspaceDirectory = Path.Combine("root", "project1");
|
|
|
|
WhenMigrating(projectDirectories, workspaceDirectory)
|
|
.RootBackupDirectory
|
|
.FullName
|
|
.Should()
|
|
.Be(new DirectoryInfo(Path.Combine("root", "backup")).FullName.EnsureTrailingSlash());
|
|
}
|
|
|
|
[Fact]
|
|
public void TheBackupDirectoryIsInTheCommonRootOfTwoProjectFoldersWhenInitiatedFromCommonRoot()
|
|
{
|
|
var projectDirectories = new []
|
|
{
|
|
Path.Combine("root", "project1"),
|
|
Path.Combine("root", "project2")
|
|
};
|
|
|
|
var workspaceDirectory = Path.Combine("root");
|
|
|
|
WhenMigrating(projectDirectories, workspaceDirectory)
|
|
.RootBackupDirectory
|
|
.FullName
|
|
.Should()
|
|
.Be(new DirectoryInfo(Path.Combine("root", "backup")).FullName.EnsureTrailingSlash());
|
|
}
|
|
|
|
[Fact]
|
|
public void TheBackupDirectoryIsInTheCommonRootOfTwoProjectFoldersAtDifferentLevelsWhenInitiatedFromProjectFolder()
|
|
{
|
|
var projectDirectories = new []
|
|
{
|
|
Path.Combine("root", "tests", "inner", "project1"),
|
|
Path.Combine("root", "src", "project2")
|
|
};
|
|
|
|
var workspaceDirectory = Path.Combine("root", "tests", "inner");
|
|
|
|
WhenMigrating(projectDirectories, workspaceDirectory)
|
|
.RootBackupDirectory
|
|
.FullName
|
|
.Should()
|
|
.Be(new DirectoryInfo(Path.Combine("root", "backup")).FullName.EnsureTrailingSlash());
|
|
}
|
|
|
|
[Fact]
|
|
public void FilesToBackUpAreIdentifiedInTheRootProjectDirectory()
|
|
{
|
|
var workspaceDirectory = Path.Combine("src", "root");
|
|
var projectDirectory = Path.Combine("src", "root");
|
|
|
|
var whenMigrating = WhenMigrating(projectDirectory, workspaceDirectory);
|
|
|
|
whenMigrating
|
|
.FilesToMove(whenMigrating.ProjectBackupDirectories.Single())
|
|
.Should()
|
|
.Contain(_ => _.FullName == Path.Combine(new DirectoryInfo(workspaceDirectory).FullName, "project.json"));
|
|
}
|
|
|
|
[Fact]
|
|
public void FilesToBackUpAreIdentifiedInTheDependencyProjectDirectory()
|
|
{
|
|
var workspaceDirectory = Path.Combine("src", "root");
|
|
var projectDirectory = Path.Combine("src", "root");
|
|
|
|
var whenMigrating = WhenMigrating(projectDirectory, workspaceDirectory);
|
|
|
|
whenMigrating
|
|
.FilesToMove(whenMigrating.ProjectBackupDirectories.Single())
|
|
.Should()
|
|
.Contain(_ => _.FullName == Path.Combine(new DirectoryInfo(projectDirectory).FullName, "project.json"));
|
|
}
|
|
|
|
private MigrationBackupPlan WhenMigrating(string projectDirectory, string workspaceDirectory) =>
|
|
new MigrationBackupPlan(
|
|
new [] { new DirectoryInfo(projectDirectory) },
|
|
new DirectoryInfo(workspaceDirectory),
|
|
dir => new [] { new FileInfo(Path.Combine(dir.FullName, "project.json")) });
|
|
|
|
private MigrationBackupPlan WhenMigrating(string[] projectDirectories, string workspaceDirectory) =>
|
|
new MigrationBackupPlan(
|
|
projectDirectories.Select(p => new DirectoryInfo(p)),
|
|
new DirectoryInfo(workspaceDirectory),
|
|
dir => new [] { new FileInfo(Path.Combine(dir.FullName, "project.json")) });
|
|
}
|
|
}
|