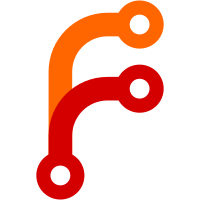
Previously, Razor server discovery for the `build-server shutdown` command was implemented by invoking MSBuild on a project file in the current directory to evaluate the path to the Razor server dll. This was problematic since it would only discover a single running Razor server instance and required that the user run the `build-server shutdown` command from a specific location. Razor's server now writes a "pid file" to a well-known location (`~/.dotnet/pids/build`) which the command can now enumerate to discover, and shutdown, the running Razor servers. This commit changes the Razor server discovery to use the pid files and removes the requirement that users need to run the command in specific directories to work. Fixes #9084.
63 lines
2.3 KiB
C#
63 lines
2.3 KiB
C#
// Copyright (c) .NET Foundation and contributors. All rights reserved.
|
|
// Licensed under the MIT license. See LICENSE file in the project root for full license information.
|
|
|
|
using System;
|
|
using System.Linq;
|
|
using FluentAssertions;
|
|
using Microsoft.DotNet.BuildServer;
|
|
using Microsoft.DotNet.Cli;
|
|
using Microsoft.DotNet.Cli.Utils;
|
|
using Microsoft.DotNet.Tools;
|
|
using Microsoft.Extensions.EnvironmentAbstractions;
|
|
using Moq;
|
|
using NuGet.Frameworks;
|
|
using Xunit;
|
|
using LocalizableStrings = Microsoft.DotNet.BuildServer.LocalizableStrings;
|
|
|
|
namespace Microsoft.DotNet.Tests.BuildServerTests
|
|
{
|
|
public class VBCSCompilerServerTests
|
|
{
|
|
[Fact]
|
|
public void GivenAZeroExitShutdownDoesNotThrow()
|
|
{
|
|
var server = new VBCSCompilerServer(CreateCommandFactoryMock().Object);
|
|
server.Shutdown();
|
|
}
|
|
|
|
[Fact]
|
|
public void GivenANonZeroExitCodeShutdownThrows()
|
|
{
|
|
const string ErrorMessage = "failed!";
|
|
|
|
var server = new VBCSCompilerServer(CreateCommandFactoryMock(exitCode: 1, stdErr: ErrorMessage).Object);
|
|
|
|
Action a = () => server.Shutdown();
|
|
|
|
a.ShouldThrow<BuildServerException>().WithMessage(
|
|
string.Format(
|
|
LocalizableStrings.ShutdownCommandFailed,
|
|
ErrorMessage));
|
|
}
|
|
|
|
private Mock<ICommandFactory> CreateCommandFactoryMock(int exitCode = 0, string stdErr = "")
|
|
{
|
|
var commandMock = new Mock<ICommand>(MockBehavior.Strict);
|
|
commandMock.Setup(c => c.CaptureStdOut()).Returns(commandMock.Object);
|
|
commandMock.Setup(c => c.CaptureStdErr()).Returns(commandMock.Object);
|
|
commandMock.Setup(c => c.Execute()).Returns(new CommandResult(null, exitCode, "", stdErr));
|
|
|
|
var commandFactoryMock = new Mock<ICommandFactory>(MockBehavior.Strict);
|
|
commandFactoryMock
|
|
.Setup(
|
|
f => f.Create(
|
|
"exec",
|
|
new string[] { VBCSCompilerServer.VBCSCompilerPath, "-shutdown" },
|
|
It.IsAny<NuGetFramework>(),
|
|
Constants.DefaultConfiguration))
|
|
.Returns(commandMock.Object);
|
|
|
|
return commandFactoryMock;
|
|
}
|
|
}
|
|
}
|