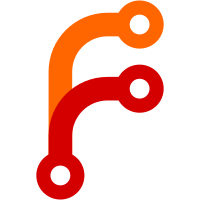
It is not currently possible when there is a -f|--framework argument because we cannot force a TargetFramework global property on to the restore evaluation. Doing so completely breaks restore by applying the TargetFramework to all projects transitively. The correct behavior is to restore for all frameworks, then build/publish/etc for the given target framework. Achieving that still requires two distinct msbuild invocations. This also changes the verbosity of implicit restore from quiet to that of the subsequent command (default=minimal). Similar to global properties, we cannot specify a distinct console verbosity for the /restore portion of the overall execution. For consistency, we apply the same verbosity change to the case where we still use two separate msbuild invocations. This also fixes an issue where the separate restore invocation's msbuild log would be overwritten by the subsequent command execution. However, this remains unfixed in the case where we still use two separate msbuild invocations.
42 lines
2.1 KiB
C#
42 lines
2.1 KiB
C#
// Copyright (c) .NET Foundation and contributors. All rights reserved.
|
|
// Licensed under the MIT license. See LICENSE file in the project root for full license information.
|
|
|
|
using Microsoft.DotNet.Tools.Pack;
|
|
using FluentAssertions;
|
|
using Xunit;
|
|
using System;
|
|
using System.Linq;
|
|
|
|
namespace Microsoft.DotNet.Cli.MSBuild.Tests
|
|
{
|
|
public class GivenDotnetPackInvocation
|
|
{
|
|
const string ExpectedPrefix = "exec <msbuildpath> /m /v:m /restore /t:pack";
|
|
|
|
[Theory]
|
|
[InlineData(new string[] { }, "")]
|
|
[InlineData(new string[] { "-o", "<packageoutputpath>" }, "/p:PackageOutputPath=<packageoutputpath>")]
|
|
[InlineData(new string[] { "--output", "<packageoutputpath>" }, "/p:PackageOutputPath=<packageoutputpath>")]
|
|
[InlineData(new string[] { "--no-build" }, "/p:NoBuild=true")]
|
|
[InlineData(new string[] { "--include-symbols" }, "/p:IncludeSymbols=true")]
|
|
[InlineData(new string[] { "--include-source" }, "/p:IncludeSource=true")]
|
|
[InlineData(new string[] { "-c", "<config>" }, "/p:Configuration=<config>")]
|
|
[InlineData(new string[] { "--configuration", "<config>" }, "/p:Configuration=<config>")]
|
|
[InlineData(new string[] { "--version-suffix", "<versionsuffix>" }, "/p:VersionSuffix=<versionsuffix>")]
|
|
[InlineData(new string[] { "-s" }, "/p:Serviceable=true")]
|
|
[InlineData(new string[] { "--serviceable" }, "/p:Serviceable=true")]
|
|
[InlineData(new string[] { "-v", "diag" }, "/verbosity:diag")]
|
|
[InlineData(new string[] { "--verbosity", "diag" }, "/verbosity:diag")]
|
|
[InlineData(new string[] { "<project>" }, "<project>")]
|
|
public void MsbuildInvocationIsCorrect(string[] args, string expectedAdditionalArgs)
|
|
{
|
|
expectedAdditionalArgs = (string.IsNullOrEmpty(expectedAdditionalArgs) ? "" : $" {expectedAdditionalArgs}");
|
|
|
|
var msbuildPath = "<msbuildpath>";
|
|
var command = PackCommand.FromArgs(args, msbuildPath);
|
|
|
|
command.SeparateRestoreCommand.Should().BeNull();
|
|
command.GetProcessStartInfo().Arguments.Should().Be($"{ExpectedPrefix}{expectedAdditionalArgs}");
|
|
}
|
|
}
|
|
}
|