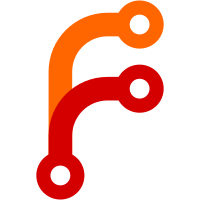
Currently, dotnet will crash with an `ArgumentNullException` if `USERPROFILE` (Windows) or `HOME` (macOS and Linux) is not set in the environment. This is because there is a missing null check after retrieving the environment variable's value. Additionally, if either variable is set to an empty string, a `.dotnet` directory is created in the current directory where dotnet is being run. This commit fixes this by printing a graceful error informing the user the home directory could not be determined and to set `DOTNET_CLI_HOME` to the directory to use. This variable will be respected before `USERPROFILE` or `HOME`. It is likely that CI environments where `HOME` is not set can use `DOTNET_CLI_HOME` to specify a local temporary location; by using this variable rather than setting `HOME`, it is guaranteed to only affect dotnet. It was discussed that we should perhaps fallback to some temporary location if the home directory could not be determined, but NuGet currently requires `HOME` to be set to work. Because of this, it was decided that we should just handle this case gracefully and provide a way for users to override the home directory without relying on `USERPROFILE`/`HOME` entirely. Closes #8053.
53 lines
1.9 KiB
C#
53 lines
1.9 KiB
C#
// Copyright (c) .NET Foundation and contributors. All rights reserved.
|
||
// Licensed under the MIT license. See LICENSE file in the project root for full license information.
|
||
|
||
using System;
|
||
using System.IO;
|
||
using FluentAssertions;
|
||
using Microsoft.DotNet.Cli.Utils;
|
||
using Microsoft.DotNet.Configurer;
|
||
using Microsoft.DotNet.Tools.Test.Utilities;
|
||
using Xunit;
|
||
|
||
using LocalizableStrings = Microsoft.DotNet.Cli.Utils.LocalizableStrings;
|
||
|
||
namespace Microsoft.DotNet.Tests
|
||
{
|
||
public class GivenThatDotNetRunsCommands : TestBase
|
||
{
|
||
[Fact]
|
||
public void UnresolvedPlatformReferencesFailAsExpected()
|
||
{
|
||
var testInstance = TestAssets.Get("NonRestoredTestProjects", "TestProjectWithUnresolvedPlatformDependency")
|
||
.CreateInstance()
|
||
.WithSourceFiles()
|
||
.Root.FullName;
|
||
|
||
new RestoreCommand()
|
||
.WithWorkingDirectory(testInstance)
|
||
.ExecuteWithCapturedOutput("/p:SkipInvalidConfigurations=true")
|
||
.Should()
|
||
.Fail();
|
||
|
||
new DotnetCommand()
|
||
.WithWorkingDirectory(testInstance)
|
||
.ExecuteWithCapturedOutput("crash")
|
||
.Should().Fail()
|
||
.And.HaveStdErrContaining(string.Format(LocalizableStrings.NoExecutableFoundMatchingCommand, "dotnet-crash"));
|
||
}
|
||
|
||
[Theory]
|
||
[InlineData("")]
|
||
[InlineData(null)]
|
||
public void GivenAMissingHomeVariableItPrintsErrorMessage(string value)
|
||
{
|
||
new TestCommand("dotnet")
|
||
.WithEnvironmentVariable(CliFolderPathCalculator.PlatformHomeVariableName, value)
|
||
.ExecuteWithCapturedOutput("--help")
|
||
.Should()
|
||
.Fail()
|
||
.And
|
||
.HaveStdErrContaining(CliFolderPathCalculator.DotnetHomeVariableName);
|
||
}
|
||
}
|
||
}
|