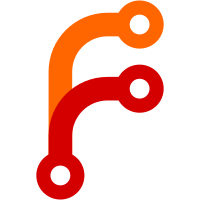
* Move dotnet-new templates to Sdk attribute * Update to MSBuild 15.1.0-preview-000454-01 To pick up a fix for Microsoft/msbuild#1431. * Fix template newlines * Fix casing on Microsoft.Net.Sdk * Move migration test csproj's to Sdk attribute * Disable parallel sdk restore Each SDK restore operation will try to manipulate the same assets.json file since the dependency name&version are injected into a common csproj file. This can cause runtime failures when two NuGets try to restore the project at once. * Make casing of SDK 'NET' and not 'Net' * Remove redundatn imports * Fix test string * Additional race * Replacing the SDK with the Web.Sdk when it is a Web project. * Fixing the test by writting the csproj before running the migration rule.
64 lines
No EOL
2.3 KiB
C#
64 lines
No EOL
2.3 KiB
C#
// Copyright (c) .NET Foundation and contributors. All rights reserved.
|
|
// Licensed under the MIT license. See LICENSE file in the project root for full license information.
|
|
|
|
using Microsoft.Build.Construction;
|
|
using Microsoft.DotNet.Tools.Test.Utilities;
|
|
using System.Linq;
|
|
using Xunit;
|
|
using FluentAssertions;
|
|
using Microsoft.DotNet.ProjectJsonMigration;
|
|
using Microsoft.DotNet.ProjectJsonMigration.Rules;
|
|
using System;
|
|
using System.IO;
|
|
|
|
namespace Microsoft.DotNet.ProjectJsonMigration.Tests
|
|
{
|
|
public class GivenThatIWantToMigrateWebProjects : PackageDependenciesTestBase
|
|
{
|
|
[Fact]
|
|
public void ItMigratesWebProjectsToHaveWebSdkInTheSdkAttribute()
|
|
{
|
|
var csprojFilePath = RunMigrateWebSdkRuleOnPj(@"
|
|
{
|
|
""buildOptions"": {
|
|
""emitEntryPoint"": true
|
|
},
|
|
""dependencies"": {
|
|
""Microsoft.AspNetCore.Mvc"" : {
|
|
""version"": ""1.0.0""
|
|
}
|
|
},
|
|
""frameworks"": {
|
|
""netcoreapp1.0"": {}
|
|
}
|
|
}");
|
|
|
|
File.ReadAllText(csprojFilePath).Should().Contain(@"Sdk=""Microsoft.NET.Sdk.Web""");
|
|
}
|
|
|
|
private string RunMigrateWebSdkRuleOnPj(string s, string testDirectory = null)
|
|
{
|
|
testDirectory = testDirectory ?? Temp.CreateDirectory().Path;
|
|
var csprojFilePath = Path.Combine(testDirectory, $"{GetContainingFolderName(testDirectory)}.csproj");
|
|
|
|
File.WriteAllText(csprojFilePath, @"
|
|
<Project Sdk=""Microsoft.NET.Sdk"" ToolsVersion=""15.0"" xmlns=""http://schemas.microsoft.com/developer/msbuild/2003"">
|
|
<PropertyGroup />
|
|
<ItemGroup />
|
|
</Project>");
|
|
|
|
TemporaryProjectFileRuleRunner.RunRules(new IMigrationRule[]
|
|
{
|
|
new MigrateWebSdkRule()
|
|
}, s, testDirectory);
|
|
|
|
return csprojFilePath;
|
|
}
|
|
|
|
private static string GetContainingFolderName(string projectDirectory)
|
|
{
|
|
projectDirectory = projectDirectory.TrimEnd(new char[] { '/', '\\' });
|
|
return Path.GetFileName(projectDirectory);
|
|
}
|
|
}
|
|
} |