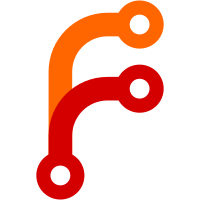
- Needs a clean machine without dotnet MSI installed for the tests to run. - Needs admin privileges to run. Else test script exits silently. - These xunit based tests run on Netfx46. For now these tests are disabled until I figure out the right way to run them in the CI machines.
45 lines
1.2 KiB
C#
45 lines
1.2 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Linq;
|
|
using Microsoft.Win32;
|
|
using Xunit;
|
|
|
|
namespace Dotnet.Cli.Msi.Tests
|
|
{
|
|
public class PostUninstallTests : InstallFixture
|
|
{
|
|
private MsiManager _msiMgr;
|
|
|
|
public PostUninstallTests()
|
|
{
|
|
_msiMgr = base.MsiManager;
|
|
}
|
|
|
|
[Fact]
|
|
public void DotnetOnPathTest()
|
|
{
|
|
Assert.True(_msiMgr.IsInstalled);
|
|
|
|
_msiMgr.UnInstall();
|
|
|
|
Assert.False(_msiMgr.IsInstalled);
|
|
Assert.False(Utils.ExistsOnPath("dotnet.exe"), "After uninstallation dotnet tools must not be on path");
|
|
}
|
|
|
|
[Fact]
|
|
public void DotnetRegKeysTest()
|
|
{
|
|
Assert.True(_msiMgr.IsInstalled);
|
|
|
|
_msiMgr.UnInstall();
|
|
|
|
Assert.False(_msiMgr.IsInstalled);
|
|
|
|
var hklm = RegistryKey.OpenBaseKey(RegistryHive.LocalMachine, RegistryView.Registry64);
|
|
Assert.Null(hklm.OpenSubKey(@"SOFTWARE\dotnet\Setup", false));
|
|
|
|
hklm = RegistryKey.OpenBaseKey(RegistryHive.LocalMachine, RegistryView.Registry32);
|
|
Assert.Null(hklm.OpenSubKey(@"SOFTWARE\dotnet\Setup", false));
|
|
}
|
|
}
|
|
}
|