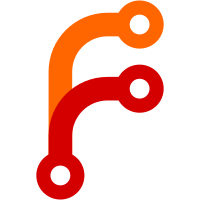
On Windows, `PathUtility.GetRelativePath` was not properly handling paths that differed by case in the drive reference (e.g. "C:\" vs. "c:\"). The fix was to add the missing case-insensitive comparison argument. Replaced uses of `PathUtility.GetRelativePath` with `Path.GetRelativePath` where possible (requires 2.0.0+). Additionally, `PathUtility.RemoveExtraPathSeparators` was not handling paths with drive references on Windows. If the path contained a drive reference, the separator between the drive reference and the first part of the path was removed. This is due to `Path.Combine` not handling this case, so an explicit concatenation of the separator was added. This commit resolves issue #7699.
42 lines
1.2 KiB
C#
42 lines
1.2 KiB
C#
// Copyright (c) .NET Foundation and contributors. All rights reserved.
|
|
// Licensed under the MIT license. See LICENSE file in the project root for full license information.
|
|
|
|
using System;
|
|
using System.IO;
|
|
using Microsoft.Build.Utilities;
|
|
using Microsoft.Build.Framework;
|
|
using Microsoft.DotNet.Cli.Build.Framework;
|
|
|
|
namespace Microsoft.DotNet.Cli.Build
|
|
{
|
|
public class MakeRelative : Task
|
|
{
|
|
[Required]
|
|
public string Path1 { get; set; }
|
|
|
|
[Required]
|
|
public string Path2 { get; set; }
|
|
|
|
[Output]
|
|
public ITaskItem RelativePath { get; set; }
|
|
|
|
public override bool Execute()
|
|
{
|
|
RelativePath = ToTaskItem(Path1, Path2, Path.GetRelativePath(Path1, Path2));
|
|
|
|
return true;
|
|
}
|
|
|
|
private static TaskItem ToTaskItem(string path1, string path2, string relativePath)
|
|
{
|
|
var framework = new TaskItem();
|
|
framework.ItemSpec = relativePath;
|
|
|
|
framework.SetMetadata("Path1", path1);
|
|
framework.SetMetadata("Path2", path2);
|
|
framework.SetMetadata("RelativePath", relativePath);
|
|
|
|
return framework;
|
|
}
|
|
}
|
|
}
|