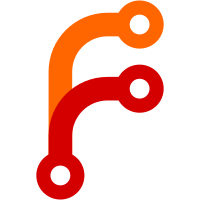
* Change to escape string via XML * tool-path option -- "Session tool" From the beginning design, shim and packageInstaller take package location from constructor and don't have assumption anymore. From previous discussion, tool-path will simply change global location to the one user want, and everything else is the same. However, this "override" need to happen during the call, that means InstallToolCommand will create different shim and packageInstaller object according to the tool-path during the call instead of constructor DI. * global package location change * block of leading dot as command name * Localization of tool-path option
70 lines
2.6 KiB
C#
70 lines
2.6 KiB
C#
// Copyright (c) .NET Foundation and contributors. All rights reserved.
|
|
// Licensed under the MIT license. See LICENSE file in the project root for full license information.
|
|
|
|
using System;
|
|
using System.Collections.Generic;
|
|
using System.IO;
|
|
using System.Linq;
|
|
using FluentAssertions;
|
|
using Microsoft.DotNet.Tools;
|
|
using NuGet.Protocol.Core.Types;
|
|
using Xunit;
|
|
|
|
namespace Microsoft.DotNet.ToolPackage.Tests
|
|
{
|
|
public class ToolConfigurationDeserializerTests
|
|
{
|
|
[Fact]
|
|
public void GivenXmlPathItShouldGetToolConfiguration()
|
|
{
|
|
ToolConfiguration toolConfiguration = ToolConfigurationDeserializer.Deserialize("DotnetToolSettingsGolden.xml");
|
|
|
|
toolConfiguration.CommandName.Should().Be("sayhello");
|
|
toolConfiguration.ToolAssemblyEntryPoint.Should().Be("console.dll");
|
|
}
|
|
|
|
[Fact]
|
|
public void GivenMalformedPathItThrows()
|
|
{
|
|
Action a = () => ToolConfigurationDeserializer.Deserialize("DotnetToolSettingsMalformed.xml");
|
|
a.ShouldThrow<ToolConfigurationException>()
|
|
.And.Message.Should()
|
|
.Contain(string.Format(CommonLocalizableStrings.ToolSettingsInvalidXml, string.Empty));
|
|
}
|
|
|
|
[Fact]
|
|
public void GivenMissingContentItThrows()
|
|
{
|
|
Action a = () => ToolConfigurationDeserializer.Deserialize("DotnetToolSettingsMissing.xml");
|
|
a.ShouldThrow<ToolConfigurationException>()
|
|
.And.Message.Should()
|
|
.Contain(CommonLocalizableStrings.ToolSettingsMissingCommandName);
|
|
}
|
|
|
|
[Fact]
|
|
public void GivenInvalidCharAsFileNameItThrows()
|
|
{
|
|
var invalidCommandName = "na\0me";
|
|
Action a = () => new ToolConfiguration(invalidCommandName, "my.dll");
|
|
a.ShouldThrow<ToolConfigurationException>()
|
|
.And.Message.Should()
|
|
.Contain(
|
|
string.Format(
|
|
CommonLocalizableStrings.ToolSettingsInvalidCommandName,
|
|
invalidCommandName,
|
|
string.Join(", ", Path.GetInvalidFileNameChars().Select(c => $"'{c}'"))));
|
|
}
|
|
|
|
[Fact]
|
|
public void GivenALeadingDotAsFileNameItThrows()
|
|
{
|
|
var invalidCommandName = ".mytool";
|
|
Action a = () => new ToolConfiguration(invalidCommandName, "my.dll");
|
|
a.ShouldThrow<ToolConfigurationException>()
|
|
.And.Message.Should()
|
|
.Contain(string.Format(
|
|
CommonLocalizableStrings.ToolSettingsInvalidLeadingDotCommandName,
|
|
invalidCommandName));
|
|
}
|
|
}
|
|
}
|