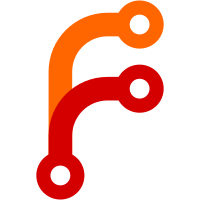
* Move dotnet-new templates to Sdk attribute * Update to MSBuild 15.1.0-preview-000454-01 To pick up a fix for Microsoft/msbuild#1431. * Fix template newlines * Fix casing on Microsoft.Net.Sdk * Move migration test csproj's to Sdk attribute * Disable parallel sdk restore Each SDK restore operation will try to manipulate the same assets.json file since the dependency name&version are injected into a common csproj file. This can cause runtime failures when two NuGets try to restore the project at once. * Make casing of SDK 'NET' and not 'Net' * Remove redundatn imports * Fix test string * Additional race * Replacing the SDK with the Web.Sdk when it is a Web project. * Fixing the test by writting the csproj before running the migration rule.
45 lines
No EOL
1.7 KiB
C#
45 lines
No EOL
1.7 KiB
C#
using System.Collections.Generic;
|
|
using Microsoft.Build.Construction;
|
|
using Microsoft.DotNet.ProjectJsonMigration.Rules;
|
|
using Microsoft.DotNet.Internal.ProjectModel;
|
|
using NuGet.Frameworks;
|
|
|
|
namespace Microsoft.DotNet.ProjectJsonMigration.Tests
|
|
{
|
|
internal class TemporaryProjectFileRuleRunner
|
|
{
|
|
public static ProjectRootElement RunRules(IEnumerable<IMigrationRule> rules, string projectJson,
|
|
string testDirectory, ProjectRootElement xproj=null)
|
|
{
|
|
var projectContext = GenerateProjectContextFromString(testDirectory, projectJson);
|
|
return RunMigrationRulesOnGeneratedProject(rules, projectContext, testDirectory, xproj);
|
|
}
|
|
|
|
private static ProjectContext GenerateProjectContextFromString(string projectDirectory, string json)
|
|
{
|
|
var testPj = new ProjectJsonBuilder(null)
|
|
.FromStringBase(json)
|
|
.SaveToDisk(projectDirectory);
|
|
|
|
return ProjectContext.Create(testPj, FrameworkConstants.CommonFrameworks.NetCoreApp10);
|
|
}
|
|
|
|
private static ProjectRootElement RunMigrationRulesOnGeneratedProject(IEnumerable<IMigrationRule> rules,
|
|
ProjectContext projectContext, string testDirectory, ProjectRootElement xproj)
|
|
{
|
|
var project = ProjectRootElement.Create();
|
|
var testSettings = new MigrationSettings(testDirectory, testDirectory, project);
|
|
var testInputs = new MigrationRuleInputs(new[] {projectContext}, project,
|
|
project.AddItemGroup(),
|
|
project.AddPropertyGroup(),
|
|
xproj);
|
|
|
|
foreach (var rule in rules)
|
|
{
|
|
rule.Apply(testSettings, testInputs);
|
|
}
|
|
|
|
return project;
|
|
}
|
|
}
|
|
} |