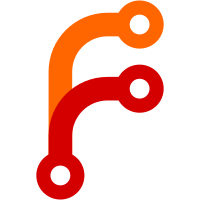
* Move dotnet-new templates to Sdk attribute * Update to MSBuild 15.1.0-preview-000454-01 To pick up a fix for Microsoft/msbuild#1431. * Fix template newlines * Fix casing on Microsoft.Net.Sdk * Move migration test csproj's to Sdk attribute * Disable parallel sdk restore Each SDK restore operation will try to manipulate the same assets.json file since the dependency name&version are injected into a common csproj file. This can cause runtime failures when two NuGets try to restore the project at once. * Make casing of SDK 'NET' and not 'Net' * Remove redundatn imports * Fix test string * Additional race * Replacing the SDK with the Web.Sdk when it is a Web project. * Fixing the test by writting the csproj before running the migration rule.
109 lines
2.9 KiB
C#
109 lines
2.9 KiB
C#
using Microsoft.DotNet.TestFramework;
|
|
using Newtonsoft.Json.Linq;
|
|
using System;
|
|
using System.Collections.Generic;
|
|
using System.IO;
|
|
using System.Linq;
|
|
using System.Threading.Tasks;
|
|
|
|
namespace Microsoft.DotNet.ProjectJsonMigration.Tests
|
|
{
|
|
/// <summary>
|
|
/// Used to build up test scenario project.jsons without needing to add a new test asset.
|
|
/// </summary>
|
|
public class ProjectJsonBuilder
|
|
{
|
|
private readonly TestAssetsManager _testAssetsManager;
|
|
private JObject _projectJson;
|
|
|
|
private bool _baseDefined = false;
|
|
|
|
public ProjectJsonBuilder(TestAssetsManager testAssetsManager)
|
|
{
|
|
_testAssetsManager = testAssetsManager;
|
|
}
|
|
|
|
public string SaveToDisk(string outputDirectory)
|
|
{
|
|
EnsureBaseIsSet();
|
|
|
|
var projectPath = Path.Combine(outputDirectory, "project.json");
|
|
File.WriteAllText(projectPath, _projectJson.ToString());
|
|
return projectPath;
|
|
}
|
|
|
|
public JObject Build()
|
|
{
|
|
EnsureBaseIsSet();
|
|
return _projectJson;
|
|
}
|
|
|
|
public ProjectJsonBuilder FromTestAssetBase(string testAssetName)
|
|
{
|
|
var testProjectDirectory = _testAssetsManager.CreateTestInstance(testAssetName).Path;
|
|
var testProject = Path.Combine(testProjectDirectory, "project.json");
|
|
|
|
SetBase(JObject.Parse(File.ReadAllText(testProject)));
|
|
|
|
return this;
|
|
}
|
|
|
|
public ProjectJsonBuilder FromStringBase(string jsonString)
|
|
{
|
|
SetBase(JObject.Parse(jsonString));
|
|
return this;
|
|
}
|
|
|
|
public ProjectJsonBuilder FromEmptyBase()
|
|
{
|
|
SetBase(new JObject());
|
|
return this;
|
|
}
|
|
|
|
public ProjectJsonBuilder WithCustomProperty(string propertyName, Dictionary<string, string> value)
|
|
{
|
|
EnsureBaseIsSet();
|
|
|
|
_projectJson[propertyName] = JObject.FromObject(value);
|
|
|
|
return this;
|
|
}
|
|
|
|
public ProjectJsonBuilder WithCustomProperty(string propertyName, string value)
|
|
{
|
|
EnsureBaseIsSet();
|
|
|
|
_projectJson[propertyName] = value;
|
|
|
|
return this;
|
|
}
|
|
|
|
public ProjectJsonBuilder WithCustomProperty(string propertyName, string[] value)
|
|
{
|
|
EnsureBaseIsSet();
|
|
|
|
_projectJson[propertyName] = JArray.FromObject(value);
|
|
|
|
return this;
|
|
}
|
|
|
|
private void SetBase(JObject project)
|
|
{
|
|
if (_baseDefined)
|
|
{
|
|
throw new Exception("Base was already defined.");
|
|
}
|
|
_baseDefined = true;
|
|
|
|
_projectJson = project;
|
|
}
|
|
|
|
private void EnsureBaseIsSet()
|
|
{
|
|
if (!_baseDefined)
|
|
{
|
|
throw new Exception("Cannot build without base set");
|
|
}
|
|
}
|
|
}
|
|
}
|