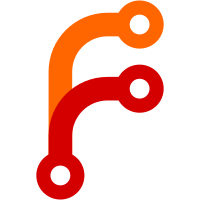
Previously, running reboot-mode as an unprivileged user resulted in Error: No error information without a newline at the end. According to SYSCALL(2), the return value of -1 indicated an error, but the actual error code is stored in errno.
51 lines
1 KiB
C
51 lines
1 KiB
C
// SPDX-License-Identifier: GPL-3.0-or-later
|
|
/*
|
|
* Reboot the device to a specific mode
|
|
*
|
|
* Copyright (C) 2019 Daniele Debernardi <drebrez@gmail.com>
|
|
*/
|
|
#include <errno.h>
|
|
#include <linux/reboot.h>
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
#include <sys/syscall.h>
|
|
#include <unistd.h>
|
|
|
|
void usage(char *appname)
|
|
{
|
|
printf("Usage: %s [-h] MODE\n\n", appname);
|
|
printf("Reboot the device to the MODE specified (e.g. recovery, bootloader)\n\n");
|
|
printf("WARNING: the reboot is instantaneous\n\n");
|
|
printf("optional arguments:\n");
|
|
printf(" -h Show this help message and exit\n");
|
|
}
|
|
|
|
int main(int argc, char **argv)
|
|
{
|
|
if (argc != 2) {
|
|
usage(argv[0]);
|
|
exit(1);
|
|
}
|
|
|
|
int opt;
|
|
while ((opt = getopt(argc, argv, "h")) != -1) {
|
|
switch (opt) {
|
|
case 'h':
|
|
default:
|
|
usage(argv[0]);
|
|
exit(1);
|
|
}
|
|
}
|
|
|
|
sync();
|
|
|
|
int ret;
|
|
ret = syscall(__NR_reboot, LINUX_REBOOT_MAGIC1, LINUX_REBOOT_MAGIC2, LINUX_REBOOT_CMD_RESTART2, argv[1]);
|
|
|
|
if (ret < 0) {
|
|
printf("Error: %s\n", strerror(errno));
|
|
}
|
|
|
|
return ret;
|
|
}
|